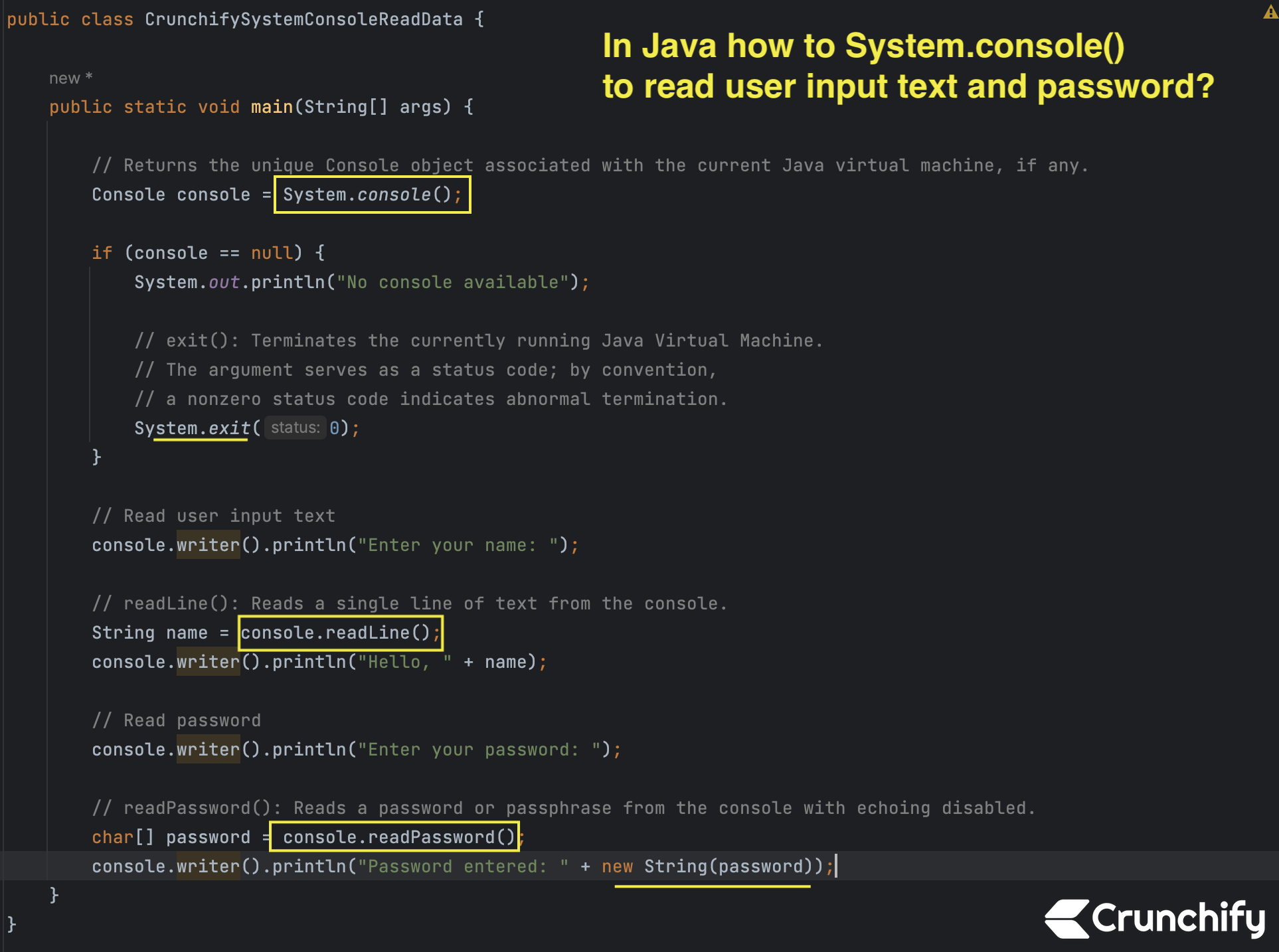
The password read using System.console().readPassword()
can be used for various purposes, such as authentication or encryption.
For example, you can use the password to check if it matches a stored password in a database or a file. If the passwords match, the user is authenticated. You can also use the password to encrypt sensitive information before storing it in a file or a database.
It is important to keep in mind that the password entered using readPassword()
is stored in a char[]
array, which is more secure than a String
object because a char[]
array can be cleared from memory after use, while a String
object cannot be cleared from memory easily.
Here is an example of how you can use System.console()
to read both user input text and password:
package crunchify.com.java.tutorials; import java.io.Console; /** * @author Crunchify.com * Version: 1.1 * In Java how to System.console() to read user input text and password?? * */ public class CrunchifySystemConsoleReadData { public static void main(String[] args) { // Returns the unique Console object associated with the current Java virtual machine, if any. Console console = System.console(); if (console == null) { System.out.println("No console available"); // exit(): Terminates the currently running Java Virtual Machine. // The argument serves as a status code; by convention, // a nonzero status code indicates abnormal termination. System.exit(0); } // Read user input text console.writer().println("Enter your name: "); // readLine(): Reads a single line of text from the console. String name = console.readLine(); console.writer().println("Hello, " + name); // Read password console.writer().println("Enter your password: "); // readPassword(): Reads a password or passphrase from the console with echoing disabled. char[] password = console.readPassword(); console.writer().println("Password entered: " + new String(password)); } }
Explanation:
- The
System.console()
method returns ajava.io.Console
object that can be used to read input from the console. - The
if
statement is used to check if the console is available, asSystem.console()
may returnnull
in some cases (for example, when the application is not run from the command line). - The
readLine()
method is used to read user input text, and thereadPassword()
method is used to read a password. - The password entered by the user is not displayed on the console, but it is stored in a
char[]
array. To display it, we convert it to aString
object.
Let me know if you have any question running this code.
The post In Java how to use System.console() to read user input text and password? appeared first on Crunchify.